本文主要是介绍【LeapMotion】Leap Motion C++配置/获取显示/显示并对齐手指,希望对大家解决编程问题提供一定的参考价值,需要的开发者们随着小编来一起学习吧!
Leap Motion 用EmguCV 显示图像并对齐手指
2015-11-03 21:39 阅读(334)
Leap Motion 使用OpenCV获取和显示图像
2015-11-01 00:10 阅读(593)
===================================================================================
Leap Motion C++环境的配置
先是建一个c++的win32项目
然后配置项目的包含目录和库目录
包含目录中添加
C:\Users\chengk\Documents\LeapDeveloperKit_2.3.0+31542_win\LeapSDK\include
当然,路径要改为你自己的。
然后在库目录中添加:
C:\Users\chengk\Documents\LeapDeveloperKit_2.3.0+31542_win\LeapSDK\lib\x86
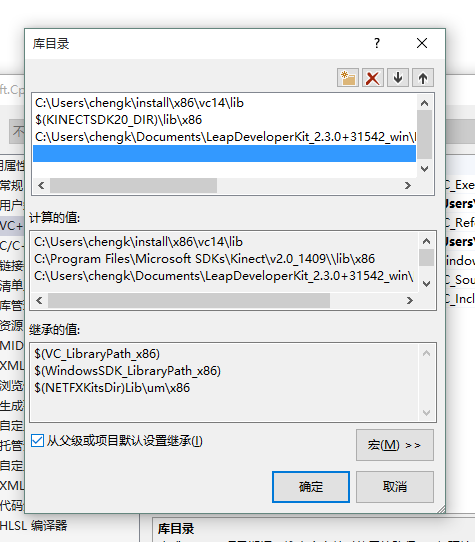
接下来级可以开始写代码了:
#include <iostream>
#include <stdio.h>
#include "opencv2/core.hpp"
#include "opencv2/core/utility.hpp"
#include "opencv2/core/ocl.hpp"
#include "opencv2/imgcodecs.hpp"
#include "opencv2/highgui.hpp"
#include "opencv2/features2d.hpp"
#include "opencv2/calib3d.hpp"
#include "opencv2/imgproc.hpp"
#include"opencv2/flann.hpp"
#include"opencv2/xfeatures2d.hpp"
#include"opencv2/ml.hpp"
#include"Leap.h"
#pragma comment ( lib, "Leap.lib" )
using namespace cv;
using namespace std;
using namespace cv::xfeatures2d;
using namespace cv::ml;
using namespace Leap;class SampleListener : public Listener
{
public:virtual void onConnect(const Controller&);virtual void onFrame(const Controller&);
};void SampleListener::onConnect(const Controller& controller)
{std::cout << "Connected" << std::endl;
}void SampleListener::onFrame(const Controller& controller)
{std::cout << "Frame available" << std::endl;
}int main()
{SampleListener listener;Controller leap;leap.addListener(listener);cin.get();leap.removeListener(listener);}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
这里重写了Listener类,让在Leap Motion连接时和frame可用是输出。
中间需要暂停下,防止还没开始进程就结束了。
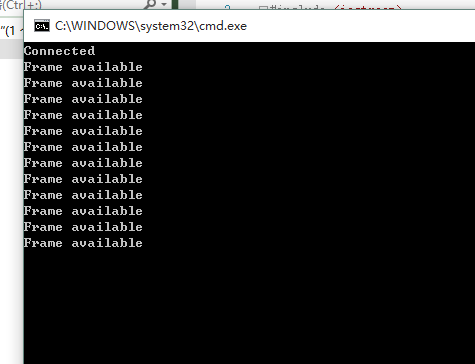
==============================================================================
Leap Motion 使用OpenCV获取和显示图像
实现的并不难,就是先设置下可以读取图像,然后在onFrame里读取下图像并显示就可以了
#define _CRT_SECURE_NO_DEPRECATE
#include <iostream>
#include <stdio.h>
#include "opencv2/core.hpp"
#include "opencv2/core/utility.hpp"
#include "opencv2/core/ocl.hpp"
#include "opencv2/imgcodecs.hpp"
#include "opencv2/highgui.hpp"
#include "opencv2/features2d.hpp"
#include "opencv2/calib3d.hpp"
#include "opencv2/imgproc.hpp"
#include"opencv2/flann.hpp"
#include"opencv2/xfeatures2d.hpp"
#include"opencv2/ml.hpp"
#include"Leap.h"
#pragma comment ( lib, "Leap.lib" )
using namespace cv;
using namespace std;
using namespace cv::xfeatures2d;
using namespace cv::ml;
using namespace Leap;class SampleListener : public Listener
{
public:virtual void onInit(const Controller&);virtual void onConnect(const Controller&);virtual void onDisconnect(const Controller&);virtual void onExit(const Controller&);virtual void onFrame(const Controller&);
};void SampleListener::onInit(const Controller& controller)
{std::cout << "Initialized" << std::endl;
}void SampleListener::onConnect(const Controller& controller)
{std::cout << "Connected" << std::endl;
}void SampleListener::onDisconnect(const Controller& controller)
{std::cout << "Disconnected" << std::endl;
}void SampleListener::onExit(const Controller& controller)
{std::cout << "Exited" << std::endl;
}void SampleListener::onFrame(const Controller& controller)
{const Frame frame = controller.frame();ImageList images = frame.images();Mat leftMat;Mat rightMat;if (images.count() == 2){leftMat = Mat(images[0].height(), images[0].width(), CV_8UC1, (void *)images[0].data());rightMat = Mat(images[1].height(), images[1].width(), CV_8UC1, (void *)images[1].data());imshow("leftMat", leftMat);imshow("rightMat", rightMat);waitKey(1);}}int main()
{SampleListener listener;Controller leap;leap.addListener(listener);leap.setPolicy(Leap::Controller::POLICY_BACKGROUND_FRAMES);leap.setPolicy(Leap::Controller::POLICY_IMAGES);std::cin.get();leap.removeListener(listener);return 0;
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
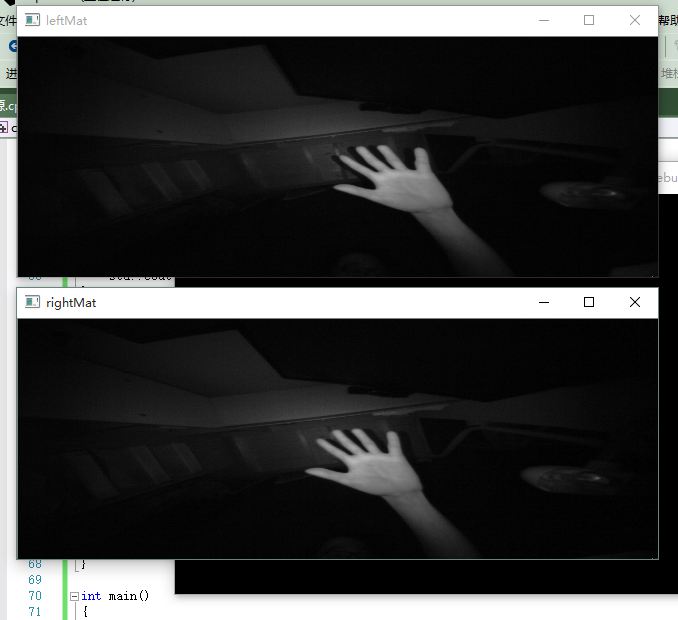
==================================================================================
Leap Motion 用EmguCV 显示图像并对齐手指
使用的为控制台应用程序。主要的代码都在重写的Listener类里,代码如下。
中间需要对坐标转换下。
我只显示了左边的那副图。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Leap;
using Emgu.CV;
using System.Drawing;
using Emgu.CV.Structure;
using Emgu.Util;
using System.Drawing.Imaging;namespace ConsoleApplication14
{class asas : Listener{Object thislock = new Object();Image<Gray, byte> left = new Image<Gray, byte>(640, 240);void safewriteline(string line){lock (thislock){Console.WriteLine(line);}}public override void OnConnect(Controller arg0){safewriteline("Conectted");}public override void OnFrame(Controller arg0){Frame frame = arg0.Frame();ImageList images = frame.Images;FingerList fingers = frame.Fingers;Image<Gray, byte> left = new Image<Gray, byte>(640,240);left.Bytes = images[0].Data;foreach(var f1 in fingers){Leap.Vector tip = f1.TipPosition;float h_slope = -(tip.x + 20 * (-1)) / tip.y;float v_slope = tip.z / tip.y;Leap.Vector pixel = images[0].Warp(new Leap.Vector(h_slope, v_slope, 0));CvInvoke.Circle(left, new Point((int)pixel.x, (int)pixel.y), (int)(1/tip.y*1000), new MCvScalar(255), (int)(1/tip.y*1000)*2);}CvInvoke.Imshow("asasa",left);CvInvoke.WaitKey(2);}public override void OnInit(Controller arg0){safewriteline("inti");}}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
然后这个是主函数的代码
namespace ConsoleApplication14
{
class MyApplication{static void Main(string[] args){Controller leap = new Controller();leap.AddListener(new asas());leap.SetPolicy(Controller.PolicyFlag.POLICY_IMAGES);Console.ReadKey();}}
}
运行图:
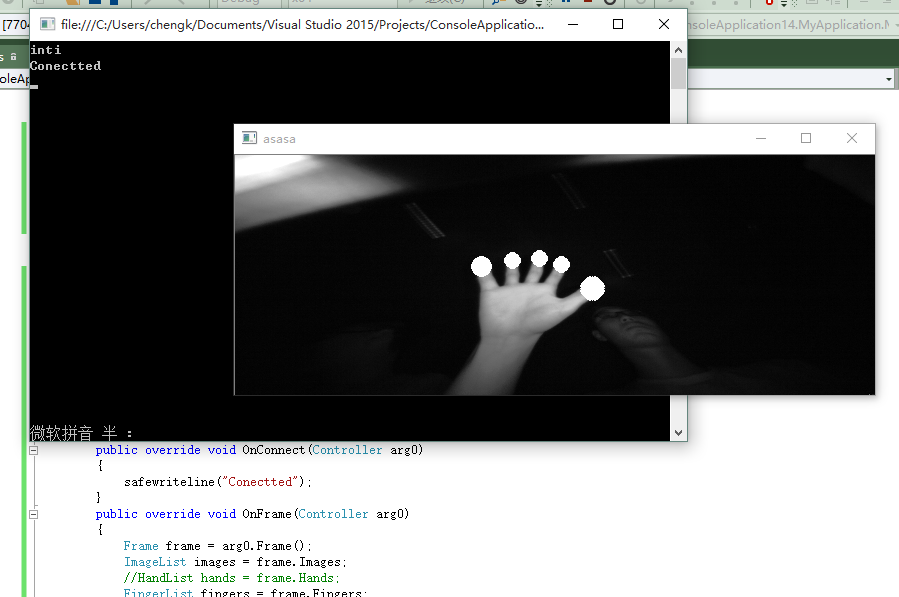
这篇关于【LeapMotion】Leap Motion C++配置/获取显示/显示并对齐手指的文章就介绍到这儿,希望我们推荐的文章对编程师们有所帮助!