本文主要是介绍cs61C | lecture4,希望对大家解决编程问题提供一定的参考价值,需要的开发者们随着小编来一起学习吧!
cs61C | lecture4
C 语言内存布局
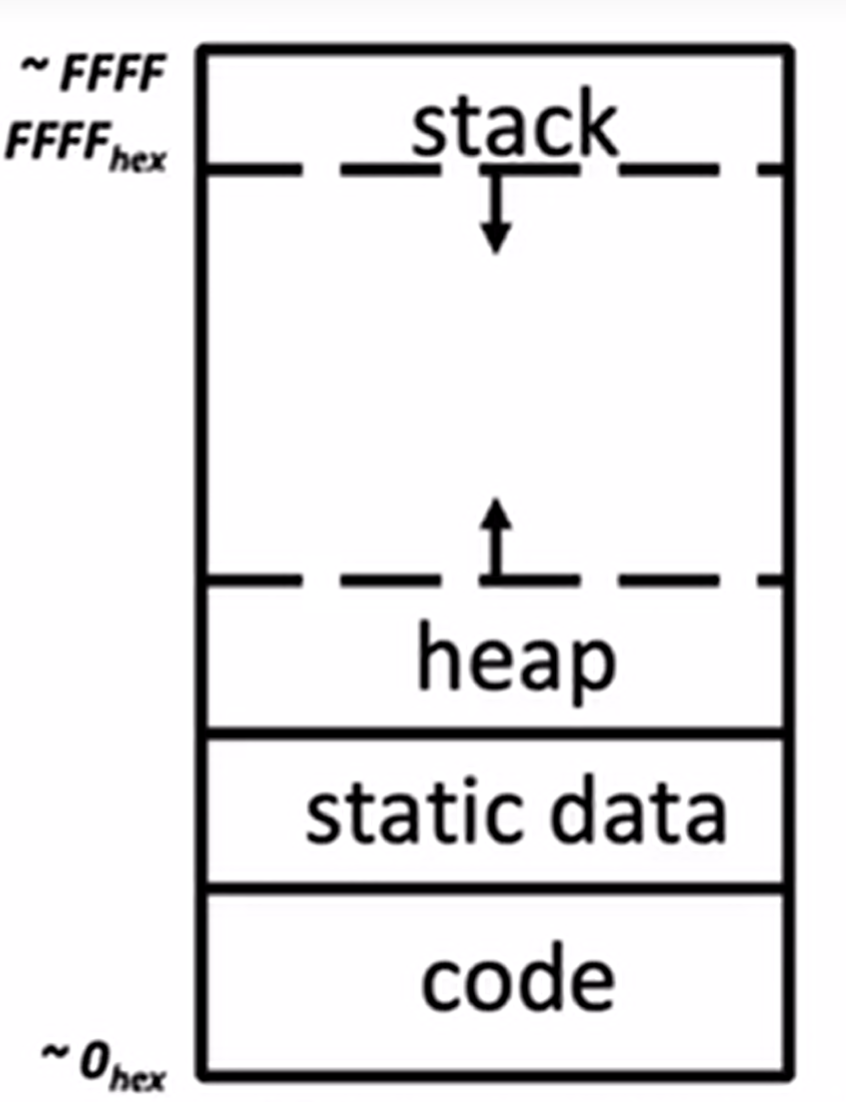
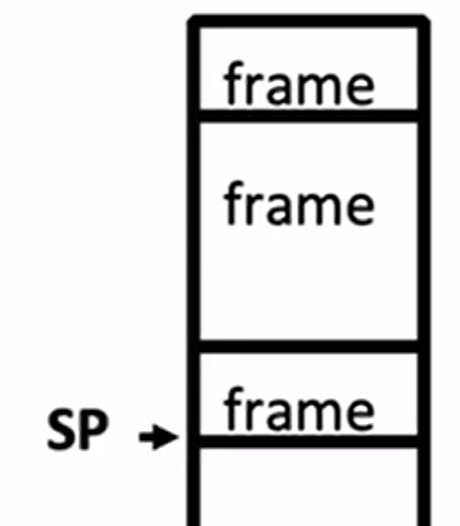
在函数外定义的变量存储在 Static Data
在函数内部声明的变量存储在 Stack,在函数返回时释放
动态分配的内存存储在 Heap
#include <stdio.h>int varGlobal; int main() {int varLocal;int *varDyn = malloc(sizeof(int));
}
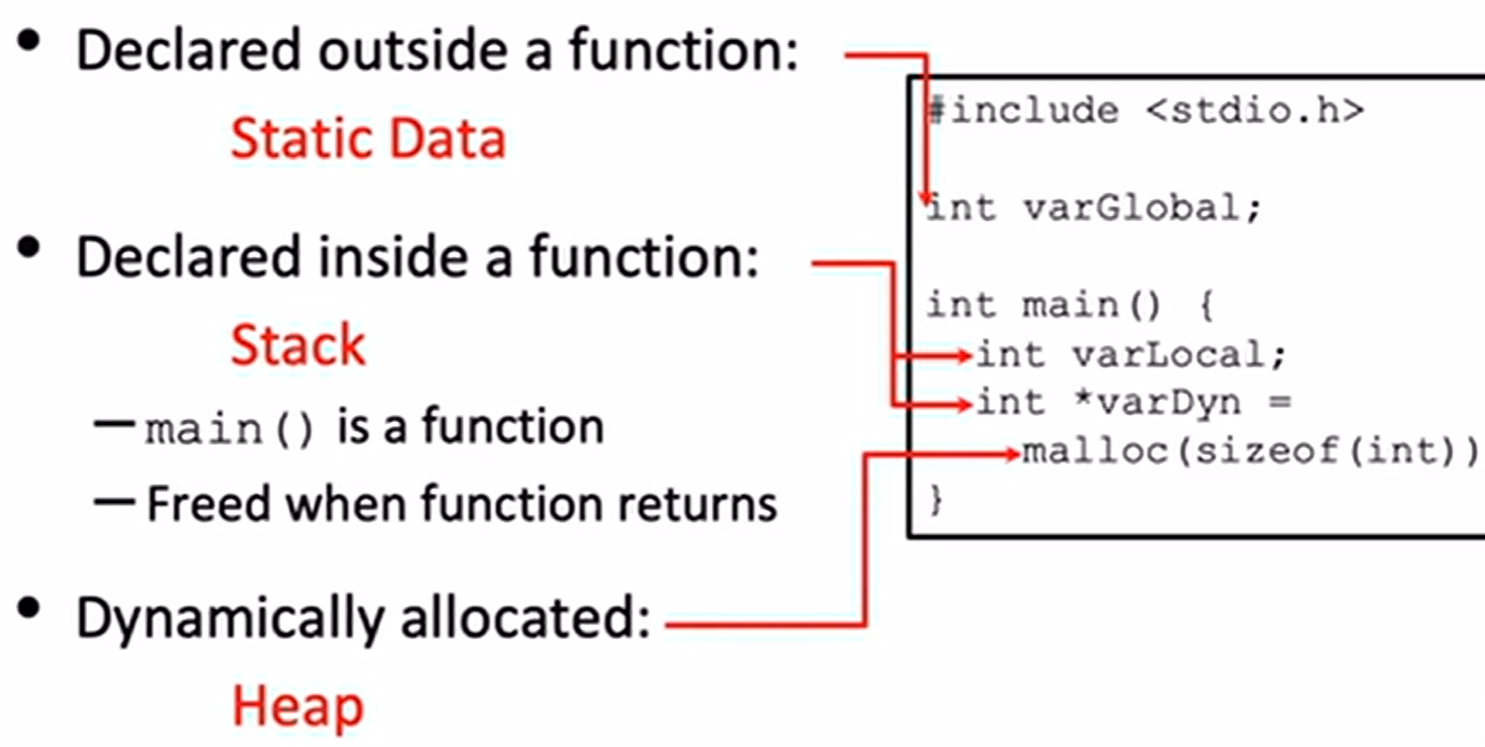
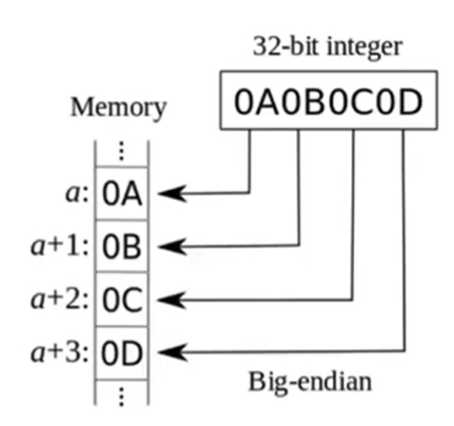
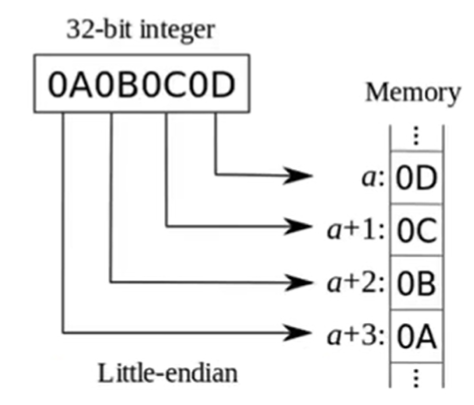
int *p = (int *)calloc(5, sizeof(int));
### 常见的内存错误
[What does the "bus error" message mean, and how does it differ from a segmentation fault?](https://stackoverflow.com/questions/212466/what-is-a-bus-error-is-it-different-from-a-segmentation-fault)
#### Segmentation Fault
尝试访问不允许的内存,比如内存访问越界、非法指针使用等。
#### Bus Error
当处理器无法尝试请求的内存访问,例如使用地址不满足对齐要求的处理器指令。
#### 使用未初始化的值
```c
void foo(int *p) {int j; /* 未初始化,是 garbage */*p = j;
}void bar() {int i = 10;foo(&i);printf("i = %d\n", i); /* i 现在包含 garbage */
}
使用不知道的内存
Using Memory You Don’t Own(1)
如果 head 为 NULL,则会引发 Seg Fault
typedef struct node {struct node* next;int val;
}Node;int findLastNodeValue(Node* head) {/* head 可能为 NULL */while(head->next != NULL) {head = head->next;}return ...
}
Using Memory You Don’t Own(2)
以下代码的问题在于该函数返回指向 result 的指针,而 result 是局部变量,在栈区创立,函数返回后该内存空间会被释放。这个指针也就指向了未知的东西。
解决方法:使用动态内存分配,用 malloc 在堆上分配内存,这样即便函数返回,内存也仍然有效。
char *append(const char* s1, const char* s2) { const int MAXSIZE = 128; char* result = (char*)malloc(MAXSIZE * sizeof(char)); // 动态分配内存 if (result == NULL) { return NULL; // 检查内存分配是否成功 }...
Using Memory You Don’t Own(3)
strlen() 函数不会算上结尾的 ‘0’。
同时也要避免双重释放,比如
free(person);
free(person->name);
Using Memory You Haven’t Allocated
实际上这被称为 BUFFER OVERRUN or BUFFER OVERFLOW。
安全的版本:
#define ARR_LEN 1024
char buffer[ARR_LEN];int foo(char *str) {strncpy(buffer, str, ARR_LEN);
}
Memory Leaks
pi 是全局变量,所以 foo() 中的 pi 覆盖了 main 函数中,main() 函数内的指针就无法被释放。
创建 Linked List
struct Node {char *value; /* 字符串 */struct Node *next; /* 指向下一个节点的指针 */
} node;
Adding a Node to the List
s1 -> s2 -> s3 -> NULL
char *s1 = "start", *s2 = "middle", *s3 = "end";
struct node *theList = NULL;
theList = addNode(s3, theList);
theList = addNode(s2, theList);
theList = addNode(s1, theList);
这些字符串实际上存储在静态区。
node *addNode(char *s, node *list) {node *new = (node *) malloc(sizeof(NodeStuct));new->value = (char *) malloc(strlen(s) + 1); /* '\0' 的存在 */strcpy(new->value, s);new->next = list;return new;
}
Removing a Node from the List
Delete/free the first node
node *deleteNode(node *list) {node *temp = list->next;free(list->value);free(list);return temp;
}
这篇关于cs61C | lecture4的文章就介绍到这儿,希望我们推荐的文章对编程师们有所帮助!