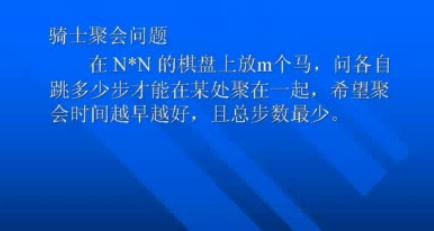
#include < stdio.h >
#include < stdlib.h >
#include < time.h >
struct point
{
int x;
int y;
int * CastStep;
};
point * GetCanGo(point, int );
void Walk(point, int );
int index = 0 ; // 当前的起点下标
int rows = 0 ;
int cells = 0 ;
point ** Chessboard;
point * StartPoint;
void main()
{
int StartNum = 0 ;
printf( " 欢迎使用神奇的棋盘^_^\n " );
printf( " 输入棋盘的行数: " );
scanf( " %d " , & rows);
printf( " 输入棋盘的列数: " );
scanf( " %d " , & cells);
printf( " 您使用了%d X %d的棋盘,输入的座标x必须在0-%d之间 y必须在0-%d之间\n " ,rows,cells,rows - 1 ,cells - 1 );
Chessboard = (point ** )malloc( 4 * rows);
for ( int e = 0 ;e < rows;e ++ )
{
Chessboard[e] = (point * )malloc( sizeof (point) * cells);
}
printf( " 请输入起点个数: " );
scanf( " %d " , & StartNum);
for ( int i = 0 ;i < rows;i ++ ) // 初始化棋盘
{
for ( int j = 0 ;j < cells;j ++ )
{
Chessboard[i][j].x = i;
Chessboard[i][j].y = j;
Chessboard[i][j].CastStep = ( int * )malloc( 4 * StartNum);
for ( int a = 0 ;a < StartNum;a ++ )
Chessboard[i][j].CastStep[a] = 0 ;
}
}
StartPoint = (point * )malloc( sizeof (point) * StartNum);
for ( int k = 0 ;k < StartNum;k ++ )
{
printf( " 输入第%d个起点的座标\nx= " ,k + 1 );
scanf( " %d " , & StartPoint[k].x);
printf( " y= " );
scanf( " %d " , & StartPoint[k].y);
}
printf( " 必须信息读取完毕!\n计算中
" );
long start = clock();
for (index = 0 ;index < StartNum;index ++ ) // 让每个起点走遍整个棋盘
{
Walk(StartPoint[index], 0 );
}
int CastSteps = 0 ;
int CastDays = 0 ;
point LastPoint;
for ( int a = 0 ;a < rows;a ++ )
{
for ( int b = 0 ;b < cells;b ++ )
{
int sum = 0 ;
int max = 0 ;
for ( int c = 0 ;c < StartNum;c ++ )
{
sum += Chessboard[a][b].CastStep[c];
if (Chessboard[a][b].CastStep[c] > max)
max = Chessboard[a][b].CastStep[c];
}
if (CastSteps >= sum && CastDays >= max)
{
CastSteps = sum;
CastDays = max;
LastPoint = Chessboard[a][b];
continue ;
}
if (CastSteps == 0 )
{
CastSteps = sum;
CastDays = max;
LastPoint = Chessboard[a][b];
}
}
}
long end = clock();
printf( " \n计算完毕,用时:%ld毫秒\n会聚地点:(%d,%d)\n需要的时间:%d天\n所有人总共花去时间:%d天\n " ,end - start,LastPoint.x,LastPoint.y,CastDays,CastSteps);
printf( " 输入任意字符退出
.. " );
char c;
scanf( " %c " , & c);
}
int count = 0 ; // 保存下一次能走的点的个数
void Walk(point nowPoint, int SumSteps)
{
Chessboard[nowPoint.x][nowPoint.y].CastStep[index] = SumSteps;
point * NextCanGo = GetCanGo(nowPoint, ++ SumSteps); // 在这里累加的注意咯
int num = count;
for ( int i = 0 ;i < num;i ++ )
{
Walk(NextCanGo[i],SumSteps);
}
}
point * GetCanGo(point nowPoint, int SumSteps) // 得到下一步可以走的点
{
count = 0 ;
point temp[ 8 ];
if ((nowPoint.x - 1 >= 0 && nowPoint.y + 2 < cells) && (Chessboard[nowPoint.x - 1 ][nowPoint.y + 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 1 ][nowPoint.y + 2 ].CastStep[index]))
{
count ++ ;
temp[ 0 ].x = nowPoint.x - 1 ;
temp[ 0 ].y = nowPoint.y + 2 ;
}
else
temp[ 0 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 1 >= 0 && nowPoint.y - 2 > 0 ) && (Chessboard[nowPoint.x - 1 ][nowPoint.y - 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 1 ][nowPoint.y - 2 ].CastStep[index]))
{
count ++ ;
temp[ 1 ].x = nowPoint.x - 1 ;
temp[ 1 ].y = nowPoint.y - 2 ;
}
else
temp[ 1 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 1 < rows && nowPoint.y + 2 < cells) && (Chessboard[nowPoint.x + 1 ][nowPoint.y + 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 1 ][nowPoint.y + 2 ].CastStep[index]))
{
count ++ ;
temp[ 2 ].x = nowPoint.x + 1 ;
temp[ 2 ].y = nowPoint.y + 2 ;
}
else
temp[ 2 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 1 < rows && nowPoint.y - 2 >= 0 ) && (Chessboard[nowPoint.x + 1 ][nowPoint.y - 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 1 ][nowPoint.y - 2 ].CastStep[index]))
{
count ++ ;
temp[ 3 ].x = nowPoint.x + 1 ;
temp[ 3 ].y = nowPoint.y - 2 ;
}
else
temp[ 3 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 2 < rows && nowPoint.y + 1 < cells) && (Chessboard[nowPoint.x + 2 ][nowPoint.y + 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 2 ][nowPoint.y + 1 ].CastStep[index]))
{
count ++ ;
temp[ 4 ].x = nowPoint.x + 2 ;
temp[ 4 ].y = nowPoint.y + 1 ;
}
else
temp[ 4 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 2 < rows && nowPoint.y - 1 >= 0 ) && (Chessboard[nowPoint.x + 2 ][nowPoint.y - 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 2 ][nowPoint.y - 1 ].CastStep[index]))
{
count ++ ;
temp[ 5 ].x = nowPoint.x + 2 ;
temp[ 5 ].y = nowPoint.y - 1 ;
}
else
temp[ 5 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 2 >= 0 && nowPoint.y + 1 < cells) && (Chessboard[nowPoint.x - 2 ][nowPoint.y + 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 2 ][nowPoint.y + 1 ].CastStep[index]))
{
count ++ ;
temp[ 6 ].x = nowPoint.x - 2 ;
temp[ 6 ].y = nowPoint.y + 1 ;
}
else
temp[ 6 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 2 >= 0 && nowPoint.y - 1 >= 0 ) && (Chessboard[nowPoint.x - 2 ][nowPoint.y - 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 2 ][nowPoint.y - 1 ].CastStep[index]))
{
count ++ ;
temp[ 7 ].x = nowPoint.x - 2 ;
temp[ 7 ].y = nowPoint.y - 1 ;
}
else
temp[ 7 ].x = - 1 ; // 为了标记用
point * re = (point * )malloc( sizeof (point) * count);
int t = 0 ;
for ( int i = 0 ;i < 8 ;i ++ )
{
if (temp[i].x != - 1 )
{
re[t] = temp[i];
t ++ ;
}
}
return re;
}
#include < stdlib.h >
#include < time.h >
struct point
{
int x;
int y;
int * CastStep;
};
point * GetCanGo(point, int );
void Walk(point, int );
int index = 0 ; // 当前的起点下标
int rows = 0 ;
int cells = 0 ;
point ** Chessboard;
point * StartPoint;
void main()
{
int StartNum = 0 ;
printf( " 欢迎使用神奇的棋盘^_^\n " );
printf( " 输入棋盘的行数: " );
scanf( " %d " , & rows);
printf( " 输入棋盘的列数: " );
scanf( " %d " , & cells);
printf( " 您使用了%d X %d的棋盘,输入的座标x必须在0-%d之间 y必须在0-%d之间\n " ,rows,cells,rows - 1 ,cells - 1 );
Chessboard = (point ** )malloc( 4 * rows);
for ( int e = 0 ;e < rows;e ++ )
{
Chessboard[e] = (point * )malloc( sizeof (point) * cells);
}
printf( " 请输入起点个数: " );
scanf( " %d " , & StartNum);
for ( int i = 0 ;i < rows;i ++ ) // 初始化棋盘
{
for ( int j = 0 ;j < cells;j ++ )
{
Chessboard[i][j].x = i;
Chessboard[i][j].y = j;
Chessboard[i][j].CastStep = ( int * )malloc( 4 * StartNum);
for ( int a = 0 ;a < StartNum;a ++ )
Chessboard[i][j].CastStep[a] = 0 ;
}
}
StartPoint = (point * )malloc( sizeof (point) * StartNum);
for ( int k = 0 ;k < StartNum;k ++ )
{
printf( " 输入第%d个起点的座标\nx= " ,k + 1 );
scanf( " %d " , & StartPoint[k].x);
printf( " y= " );
scanf( " %d " , & StartPoint[k].y);
}
printf( " 必须信息读取完毕!\n计算中


long start = clock();
for (index = 0 ;index < StartNum;index ++ ) // 让每个起点走遍整个棋盘
{
Walk(StartPoint[index], 0 );
}
int CastSteps = 0 ;
int CastDays = 0 ;
point LastPoint;
for ( int a = 0 ;a < rows;a ++ )
{
for ( int b = 0 ;b < cells;b ++ )
{
int sum = 0 ;
int max = 0 ;
for ( int c = 0 ;c < StartNum;c ++ )
{
sum += Chessboard[a][b].CastStep[c];
if (Chessboard[a][b].CastStep[c] > max)
max = Chessboard[a][b].CastStep[c];
}
if (CastSteps >= sum && CastDays >= max)
{
CastSteps = sum;
CastDays = max;
LastPoint = Chessboard[a][b];
continue ;
}
if (CastSteps == 0 )
{
CastSteps = sum;
CastDays = max;
LastPoint = Chessboard[a][b];
}
}
}
long end = clock();
printf( " \n计算完毕,用时:%ld毫秒\n会聚地点:(%d,%d)\n需要的时间:%d天\n所有人总共花去时间:%d天\n " ,end - start,LastPoint.x,LastPoint.y,CastDays,CastSteps);
printf( " 输入任意字符退出

char c;
scanf( " %c " , & c);
}
int count = 0 ; // 保存下一次能走的点的个数
void Walk(point nowPoint, int SumSteps)
{
Chessboard[nowPoint.x][nowPoint.y].CastStep[index] = SumSteps;
point * NextCanGo = GetCanGo(nowPoint, ++ SumSteps); // 在这里累加的注意咯
int num = count;
for ( int i = 0 ;i < num;i ++ )
{
Walk(NextCanGo[i],SumSteps);
}
}
point * GetCanGo(point nowPoint, int SumSteps) // 得到下一步可以走的点
{
count = 0 ;
point temp[ 8 ];
if ((nowPoint.x - 1 >= 0 && nowPoint.y + 2 < cells) && (Chessboard[nowPoint.x - 1 ][nowPoint.y + 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 1 ][nowPoint.y + 2 ].CastStep[index]))
{
count ++ ;
temp[ 0 ].x = nowPoint.x - 1 ;
temp[ 0 ].y = nowPoint.y + 2 ;
}
else
temp[ 0 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 1 >= 0 && nowPoint.y - 2 > 0 ) && (Chessboard[nowPoint.x - 1 ][nowPoint.y - 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 1 ][nowPoint.y - 2 ].CastStep[index]))
{
count ++ ;
temp[ 1 ].x = nowPoint.x - 1 ;
temp[ 1 ].y = nowPoint.y - 2 ;
}
else
temp[ 1 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 1 < rows && nowPoint.y + 2 < cells) && (Chessboard[nowPoint.x + 1 ][nowPoint.y + 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 1 ][nowPoint.y + 2 ].CastStep[index]))
{
count ++ ;
temp[ 2 ].x = nowPoint.x + 1 ;
temp[ 2 ].y = nowPoint.y + 2 ;
}
else
temp[ 2 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 1 < rows && nowPoint.y - 2 >= 0 ) && (Chessboard[nowPoint.x + 1 ][nowPoint.y - 2 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 1 ][nowPoint.y - 2 ].CastStep[index]))
{
count ++ ;
temp[ 3 ].x = nowPoint.x + 1 ;
temp[ 3 ].y = nowPoint.y - 2 ;
}
else
temp[ 3 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 2 < rows && nowPoint.y + 1 < cells) && (Chessboard[nowPoint.x + 2 ][nowPoint.y + 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 2 ][nowPoint.y + 1 ].CastStep[index]))
{
count ++ ;
temp[ 4 ].x = nowPoint.x + 2 ;
temp[ 4 ].y = nowPoint.y + 1 ;
}
else
temp[ 4 ].x = - 1 ; // 为了标记用
if ((nowPoint.x + 2 < rows && nowPoint.y - 1 >= 0 ) && (Chessboard[nowPoint.x + 2 ][nowPoint.y - 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x + 2 ][nowPoint.y - 1 ].CastStep[index]))
{
count ++ ;
temp[ 5 ].x = nowPoint.x + 2 ;
temp[ 5 ].y = nowPoint.y - 1 ;
}
else
temp[ 5 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 2 >= 0 && nowPoint.y + 1 < cells) && (Chessboard[nowPoint.x - 2 ][nowPoint.y + 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 2 ][nowPoint.y + 1 ].CastStep[index]))
{
count ++ ;
temp[ 6 ].x = nowPoint.x - 2 ;
temp[ 6 ].y = nowPoint.y + 1 ;
}
else
temp[ 6 ].x = - 1 ; // 为了标记用
if ((nowPoint.x - 2 >= 0 && nowPoint.y - 1 >= 0 ) && (Chessboard[nowPoint.x - 2 ][nowPoint.y - 1 ].CastStep[index] == 0
|| SumSteps < Chessboard[nowPoint.x - 2 ][nowPoint.y - 1 ].CastStep[index]))
{
count ++ ;
temp[ 7 ].x = nowPoint.x - 2 ;
temp[ 7 ].y = nowPoint.y - 1 ;
}
else
temp[ 7 ].x = - 1 ; // 为了标记用
point * re = (point * )malloc( sizeof (point) * count);
int t = 0 ;
for ( int i = 0 ;i < 8 ;i ++ )
{
if (temp[i].x != - 1 )
{
re[t] = temp[i];
t ++ ;
}
}
return re;
}