本文主要是介绍使用Spring Boot实现用户认证和授权,希望对大家解决编程问题提供一定的参考价值,需要的开发者们随着小编来一起学习吧!
文章目录
- 引言
- 第一章 Spring Boot概述
- 1.1 什么是Spring Boot
- 1.2 Spring Boot的主要特性
- 第二章 用户认证和授权基础知识
- 2.1 用户认证
- 2.2 用户授权
- 2.3 Spring Security概述
- 第三章 项目初始化
- 第四章 实现用户认证和授权
- 4.1 定义用户实体类和角色实体类
- 4.2 创建Repository接口
- 4.3 实现Service类
- 4.4 配置Spring Security
- 4.5 创建Controller类
- 4.6 创建Thymeleaf模板
- 第五章 部署与监控
- 5.1 部署Spring Boot应用
- 5.2 使用Docker部署Spring Boot应用
- 5.3 监控Spring Boot应用
- 结论
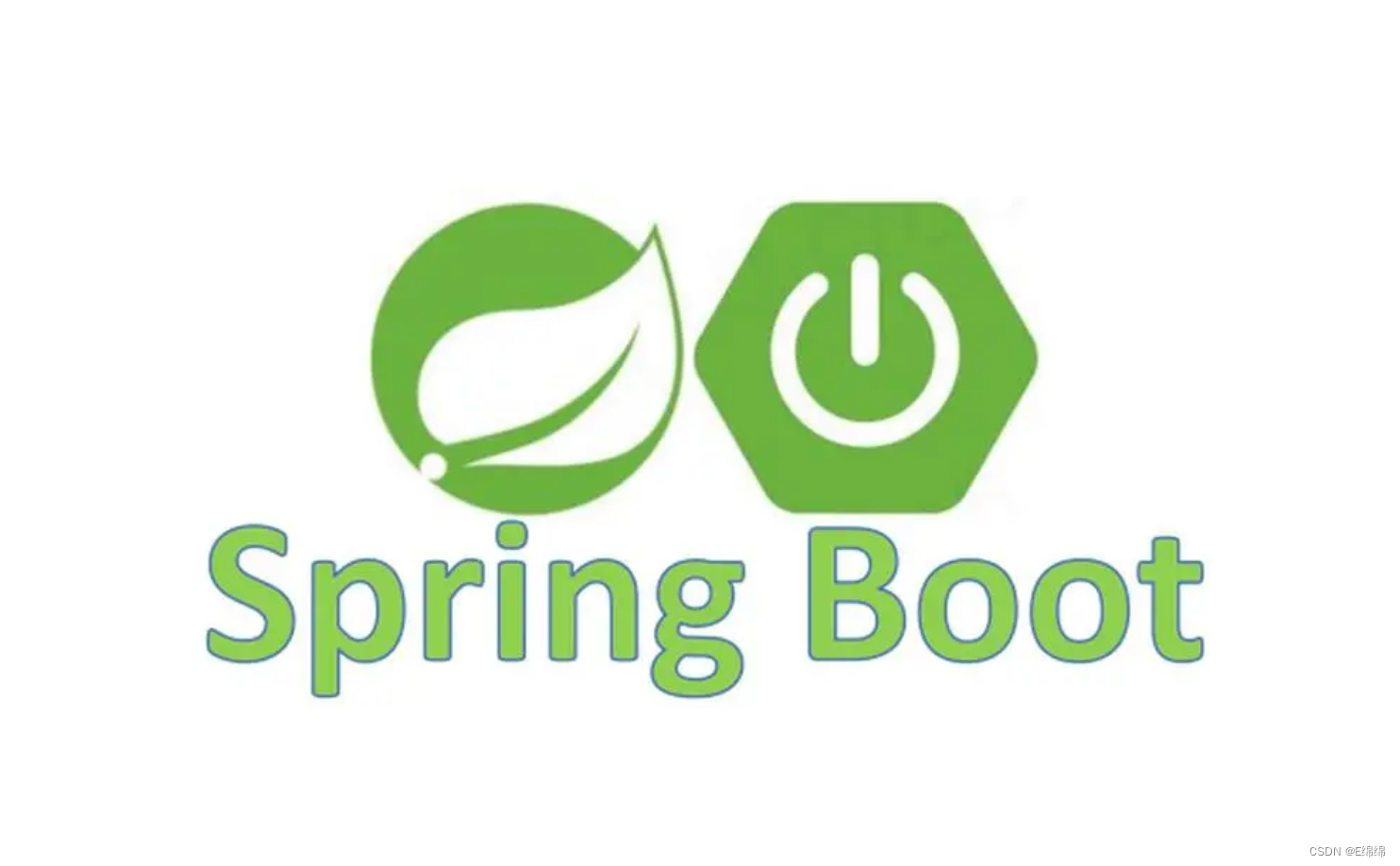
引言
在现代Web应用中,用户认证和授权是必不可少的功能。它们确保只有经过验证的用户才能访问应用,并根据用户的角色和权限进行相应的操作。Spring Boot通过集成Spring Security,提供了强大的安全功能,简化了用户认证和授权的实现。本文将详细探讨如何使用Spring Boot实现用户认证和授权,并提供具体的代码示例和应用案例。
第一章 Spring Boot概述
1.1 什么是Spring Boot
Spring Boot是一个基于Spring框架的开源项目,旨在通过简化配置和快速开发,帮助开发者构建独立、生产级的Spring应用。Spring Boot通过自动化配置、内嵌服务器和多样化的配置方式,使得开发者能够更加专注于业务逻辑,而不需要花费大量时间在繁琐的配置上。
1.2 Spring Boot的主要特性
- 自动化配置:通过自动化配置减少了大量的手动配置工作,开发者只需定义少量的配置,即可启动一个完整的Spring应用。
- 内嵌服务器:提供内嵌的Tomcat、Jetty和Undertow服务器,方便开发者在开发和测试阶段快速启动和运行应用。
- 独立运行:应用可以打包成一个可执行的JAR文件,包含所有依赖项,可以独立运行,不需要外部的应用服务器。
- 生产级功能:提供了监控、度量、健康检查等生产级功能,方便开发者管理和监控应用的运行状态。
第二章 用户认证和授权基础知识
2.1 用户认证
用户认证(Authentication)是验证用户身份的过程。常见的认证方式包括用户名和密码、OAuth、JWT等。认证的目的是确保只有合法用户才能访问系统。
2.2 用户授权
用户授权(Authorization)是对经过认证的用户进行权限控制的过程。授权决定了用户可以访问哪些资源和执行哪些操作。常见的授权方式包括基于角色的访问控制(RBAC)和基于权限的访问控制(PBAC)。
2.3 Spring Security概述
Spring Security是Spring框架的一个子项目,提供了全面的安全服务支持。Spring Security通过高度可扩展的安全机制,简化了用户认证和授权的实现。
第三章 项目初始化
使用Spring Initializr生成一个Spring Boot项目,并添加所需依赖。
<!-- 示例:通过Spring Initializr生成的pom.xml配置文件 -->
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>com.example</groupId><artifactId>auth-demo</artifactId><version>0.0.1-SNAPSHOT</version><packaging>jar</packaging><name>auth-demo</name><description>Demo project for Spring Boot Authentication and Authorization</description><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>2.5.4</version><relativePath/> <!-- lookup parent from repository --></parent><dependencies><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-security</artifactId></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-jpa</artifactId></dependency><dependency><groupId>com.h2database</groupId><artifactId>h2</artifactId><scope>runtime</scope></dependency></dependencies><build><plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId></plugin></plugins></build>
</project>
第四章 实现用户认证和授权
4.1 定义用户实体类和角色实体类
定义用户实体类和角色实体类,并配置JPA注解。
// 示例:用户实体类
package com.example.authdemo.model;import javax.persistence.*;
import java.util.HashSet;
import java.util.Set;@Entity
public class User {@Id@GeneratedValue(strategy = GenerationType.AUTO)private Long id;private String username;private String password;private boolean enabled;@ManyToMany(fetch = FetchType.EAGER)@JoinTable(name = "user_roles", joinColumns = @JoinColumn(name = "user_id"), inverseJoinColumns = @JoinColumn(name = "role_id"))private Set<Role> roles = new HashSet<>();// Getters and Setters
}// 示例:角色实体类
package com.example.authdemo.model;import javax.persistence.*;
import java.util.HashSet;
import java.util.Set;@Entity
public class Role {@Id@GeneratedValue(strategy = GenerationType.AUTO)private Long id;private String name;@ManyToMany(mappedBy = "roles")private Set<User> users = new HashSet<>();// Getters and Setters
}
4.2 创建Repository接口
创建用户和角色的JPA Repository接口,用于数据访问操作。
// 示例:用户Repository接口
package com.example.authdemo.repository;import com.example.authdemo.model.User;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;@Repository
public interface UserRepository extends JpaRepository<User, Long> {User findByUsername(String username);
}// 示例:角色Repository接口
package com.example.authdemo.repository;import com.example.authdemo.model.Role;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;@Repository
public interface RoleRepository extends JpaRepository<Role, Long> {
}
4.3 实现Service类
创建UserDetailsService实现类,处理用户认证逻辑。
// 示例:自定义UserDetailsService实现类
package com.example.authdemo.service;import com.example.authdemo.model.User;
import com.example.authdemo.repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;@Service
public class CustomUserDetailsService implements UserDetailsService {@Autowiredprivate UserRepository userRepository;@Overridepublic UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {User user = userRepository.findByUsername(username);if (user == null) {throw new UsernameNotFoundException("User not found with username: " + username);}return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), user.getRoles());}
}
4.4 配置Spring Security
配置Spring Security,实现用户认证和授权。
// 示例:Spring Security配置类
package com.example.authdemo.config;import com.example.authdemo.service.CustomUserDetailsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {@Autowiredprivate CustomUserDetailsService userDetailsService;@Overrideprotected void configure(AuthenticationManagerBuilder auth) throws Exception {auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());}@Overrideprotected void configure(HttpSecurity http) throws Exception {http.authorizeRequests().antMatchers("/admin/**").hasRole("ADMIN").antMatchers("/user/**").hasAnyRole("USER", "ADMIN").antMatchers("/", "/home", "/about").permitAll().anyRequest().authenticated().and().formLogin().loginPage("/login").permitAll().and().logout().permitAll();}@Beanpublic PasswordEncoder passwordEncoder() {return new BCryptPasswordEncoder();}
}
4.5 创建Controller类
创建控制器类,处理用户登录、注册等请求。
// 示例:用户控制器类
package com.example.authdemo.controller;import com.example.authdemo.model.User;
import com.example.authdemo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;@Controller
public class UserController {@Autowiredprivate UserService userService;@GetMapping("/login")public String login() {return "login";}@GetMapping("/register")public String register(Model model) {model.addAttribute("user", new User());return "register";}@PostMapping("/register")public String registerUser(@ModelAttribute User user) {userService.save(user);return "redirect:/login";}
}
4.6 创建Thymeleaf模板
创建Thymeleaf模板,提供用户登录和注册页面。
<!-- 示例:login.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head><title>Login</title><link rel="stylesheet" th:href="@{/css/style.css}">
</head>
<body><h1>Login</h1><form th:action="@{/login}" method="post"><div><label for="username">Username</label><input type="text" id="username" name="username" required></div><div><label for="password">Password</label><input type="password" id="password" name="password" required></div><div><button type="submit">Login</button></div></form>
</body>
</html>
<!-- 示例:register.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head><title>Register</title><link rel="stylesheet" th:href="@{/css/style.css}">
</head>
<body><h1>Register</h1><form th:action="@{/register}" method="post" th:object="${user}"><div><label for="username">Username</label><input type="text" id="username" th:field="*{username}" required></div><div><label for="password">Password</label><input type="password" id="password" th:field="*{password}" required></div><div><button type="submit">Register</button></div></form>
</body>
</html>
第五章 部署与监控
5.1 部署Spring Boot应用
Spring Boot应用可以通过多种方式进行部署,包括打包成JAR文件、Docker容器等。
# 打包Spring Boot应用
mvn clean package# 运行Spring Boot应用
java -jar target/auth-demo-0.0.1-SNAPSHOT.jar
5.2 使用Docker部署Spring Boot应用
Docker是一个开源的容器化平台,可以帮助开发者将Spring Boot应用打包成容器镜像,并在任何环境中运行。
# 示例:Dockerfile文件
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/auth-demo-0.0.1-SNAPSHOT.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
# 构建Docker镜像
docker build -t spring-boot-auth-demo .# 运行Docker容器
docker run -p 8080:8080 spring-boot-auth-demo
5.3 监控Spring Boot应用
Spring Boot Actuator提供了丰富的监控功能,通过Prometheus和Grafana,可以实现对Spring Boot应用的监控和可视化。
<!-- 示例:集成Prometheus的pom.xml配置文件 -->
<dependency><groupId>io.micrometer</groupId><artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
# 示例:Prometheus配置文件
management:endpoints:web:exposure:include: "*"endpoint:prometheus:enabled: true
结论
通过Spring Boot和Spring Security,开发者可以高效地实现用户认证和授权功能,确保系统的安全性和可靠性。本文详细介绍了用户认证和授权的基础知识、Spring Boot项目的初始化、具体实现以及部署和监控,帮助读者深入理解和掌握Spring Boot在用户认证和授权中的应用。希望本文能够为您进一步探索和应用Spring Boot提供有价值的参考。
这篇关于使用Spring Boot实现用户认证和授权的文章就介绍到这儿,希望我们推荐的文章对编程师们有所帮助!