本文主要是介绍WinForm音乐播放器_控控控-上ke控_新浪博客,希望对大家解决编程问题提供一定的参考价值,需要的开发者们随着小编来一起学习吧!
注 :当播放列表为空时会自动播放默认的背景音乐;
如:
代码如下:
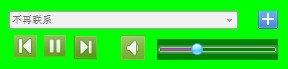
代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace Drawings_Client
{
public partial class FrmPlayer : Form
{
///
/// 当播放列表为空时默认的背景音乐
///
System.Media.SoundPlayer startSound = new System.Media.SoundPlayer(Application.StartupPath + @"\斗地主.wav");
///
/// 用于保存歌曲目录
///
string[] musicPath = new string[100]; //用于保存歌曲目录
int musicCount = 0;
public FrmPlayer()
{
InitializeComponent();
}
private void FrmPlayer_Load(object sender, EventArgs e)
{
myPlyer.BeginInit(); //初始化
myPlyer.settings.autoStart = true; //自动播放
myPlyer.settings.setMode("shuffle", false); //顺序播放
myPlyer.settings.enableErrorDialogs = true;
myPlyer.settings.balance = 0;
myPlyer.settings.mute = false;
myPlyer.settings.volume = 100; //声音设为最大
if (File.Exists("Musiclist.txt")) //如果存在播放列表,那么加载播放列表
{
StreamReader reader = new StreamReader("Musiclist.txt");
try
{
while (reader.Peek() != -1)
{
string filepath = reader.ReadLine();
if (File.Exists(filepath))
{
musicPath[musicCount++] = filepath;
string filename = Path.GetFileName(filepath).Split('.')[0];
comboBox1.Items.Add(filename); //listbox用来显示歌曲名
myPlyer.currentPlaylist.insertItem(myPlyer.currentPlaylist.count, myPlyer.newMedia(filepath));
}
}
comboBox1.SelectedIndex = 0;
}
catch (Exception)
{
comboBox1.SelectedIndex = -1;
startSound.PlayLooping();
// MessageBox.Show("加载播放列表失败或者列表为空!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
finally
{
reader.Close();
}
}
}
///
/// 音量大小条
///
///
///
private void mediaSlider1_ValueChanged(object sender, EventArgs e) //音量设置
{
myPlyer.settings.volume = mediaSlider1.Value;
}
///
/// 播放列表选择歌曲
///
///
///
private void comboBox1_SelectedValueChanged(object sender, EventArgs e) //播放列表中选中的歌曲
{
if (comboBox1.Items.Count > 0 && comboBox1.SelectedIndex >= 0)
{
startSound.Stop();
myPlyer.Ctlcontrols.playItem(myPlyer.currentPlaylist.get_Item(comboBox1.SelectedIndex));
}
}
///
/// 关闭窗体
///
///
///
private void FrmPlayer_FormClosing(object sender, FormClosingEventArgs e)
{
startSound.Stop();
myPlyer.Ctlcontrols.stop();
myPlyer.close(); //关闭播放器
StreamWriter writer = new StreamWriter("Musiclist.txt", false, Encoding.Unicode); //保存播放列表
for (int i = 0; i <= musicCount - 1; i++)
{
if (musicPath[i] != string.Empty)
{
writer.WriteLine(musicPath[i]);
}
}
writer.Close();
}
#region 窗体的按钮事件
///
/// 添加音乐或播放列表
///
///
///
private void pictureBoxAdd_Click(object sender, EventArgs e) //添加歌曲
{
DialogResult dr1 = openFileDialog1.ShowDialog(); //添加单曲
if (dr1 == DialogResult.OK)
{
string filepath = openFileDialog1.FileName;
string filename = Path.GetFileName(filepath);
comboBox1.Items.Add(filename); musicPath[musicCount++] = filepath;
myPlyer.currentPlaylist.insertItem(myPlyer.currentPlaylist.count, myPlyer.newMedia(filepath));
}
StreamWriter writer = new StreamWriter("Musiclist.txt", false, Encoding.Unicode); //保存播放列表
for (int i = 0; i <= musicCount - 1; i++)
{
if (musicPath[i] != string.Empty)
{
writer.WriteLine(musicPath[i]);
}
}
writer.Close();
//DialogResult dr = folderBrowserDialog1.ShowDialog(); //添加文件夹
//if (dr == DialogResult.OK)
//{
// string[] filepath = Directory.GetFiles(folderBrowserDialog1.SelectedPath);
// foreach (string s in filepath)
// {
// if (Path.GetExtension(s) == ".mp3")
// {
// string filename = Path.GetFileName(s);
// comboBox1.Items.Add(filename);
// musicPath[musicCount++] = s;
// myPlyer.currentPlaylist.insertItem(myPlyer.currentPlaylist.count, myPlyer.newMedia(s));
// }
// }
//}
}
///
/// 上一首
///
///
///
private void pictureBoxB_Click(object sender, EventArgs e)
{
if (comboBox1.SelectedIndex == 0)
{
comboBox1.SelectedIndex = comboBox1.Items.Count - 1;
}
else
{
comboBox1.SelectedIndex--;
}
myPlyer.Ctlcontrols.previous();
}
///
/// 播放和暂停
///
///
///
private void pictureBoxS_Click(object sender, EventArgs e)
{
if (this.toolTip1.GetToolTip(this.pictureBoxS) == "播放")//通过判断气球提示文字改变图片状态
{
startSound.Stop();
pictureBoxS.Image = Properties.Resources.b1; ;//调用资源文件图片
this.toolTip1.SetToolTip(this.pictureBoxS, "暂停");
myPlyer.Ctlcontrols.pause();
comboBox1.Enabled = true;
}
else
{
if (comboBox1.SelectedIndex != -1)
{
myPlyer.Ctlcontrols.play();
comboBox1.Enabled = false;
}
else
{
startSound.PlayLooping();
}
pictureBoxS.Image = Properties.Resources.b2;
this.toolTip1.SetToolTip(this.pictureBoxS, "播放");
}
}
///
/// 下一首
///
///
///
private void pictureBoxN_Click(object sender, EventArgs e)
{
if (comboBox1.SelectedIndex == comboBox1.Items.Count - 1)
{
comboBox1.SelectedIndex = 0;
}
else
{
comboBox1.SelectedIndex++;
}
myPlyer.Ctlcontrols.next();
}
///
/// 静音
///
///
///
private void pictureBoxY_Click(object sender, EventArgs e)
{
if (myPlyer.settings.mute == false)
{
myPlyer.settings.mute = true;
pictureBoxY.Image = Properties.Resources.d2;
}
else
{
myPlyer.settings.mute = false;
pictureBoxY.Image = Properties.Resources.d;
}
}
#endregion
}
}
这篇关于WinForm音乐播放器_控控控-上ke控_新浪博客的文章就介绍到这儿,希望我们推荐的文章对编程师们有所帮助!