本文主要是介绍JavaSE ——类和对象,希望对大家解决编程问题提供一定的参考价值,需要的开发者们随着小编来一起学习吧!
1. 面向对象
1.1 面向对象的概念
1.2 面向对象和面向过程
以洗衣服为例:
传统洗衣服:
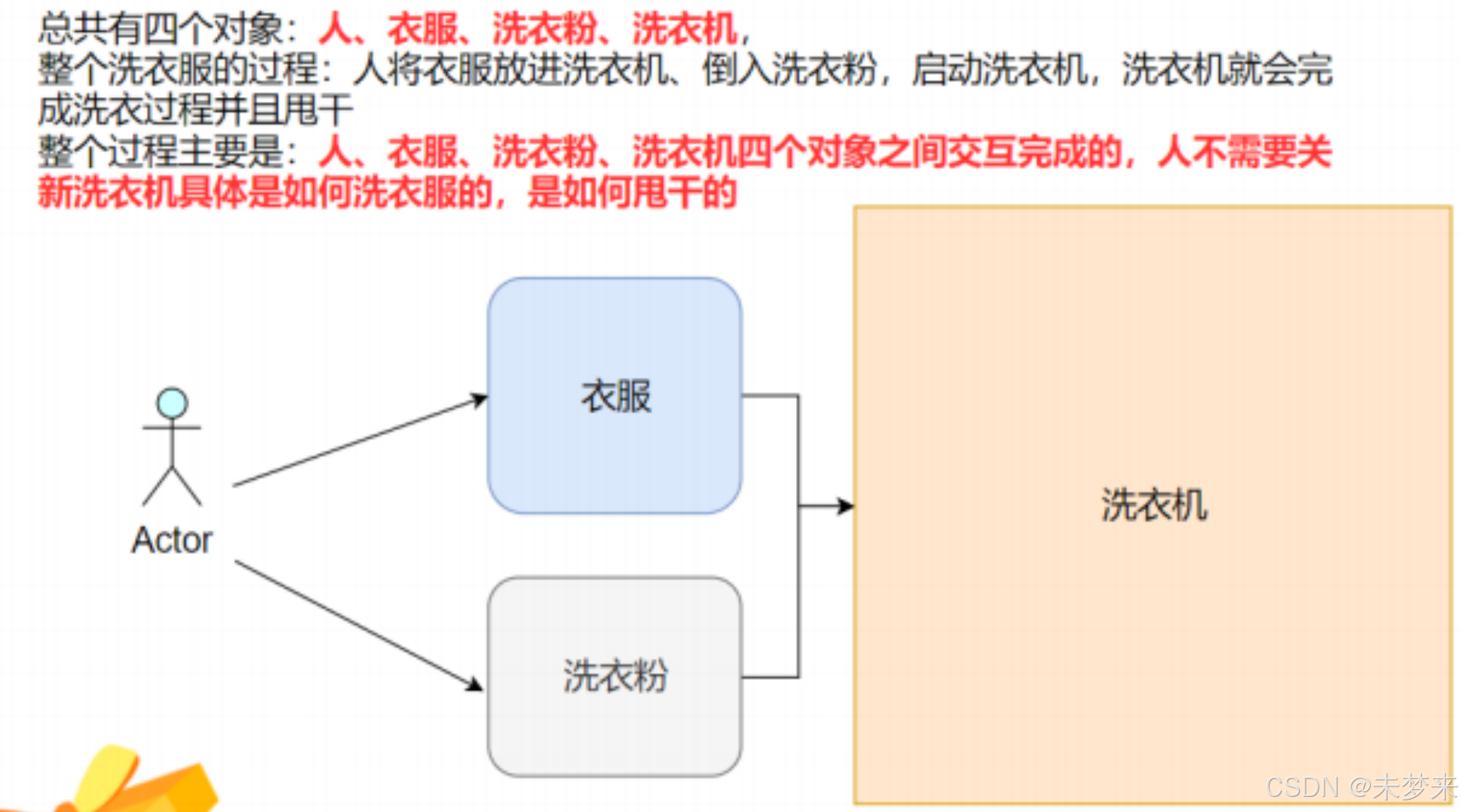
2. 类
2.1 类的定义
比如:洗衣机,它是一个品牌,在 Java 中可以将其看成是一个类别。属性:产品品牌,型号,产品重量,外观尺寸,颜色 ...功能:洗衣,烘干、定时 ....
2.2 类的定义格式
// 创建类class ClassName {field ; // 字段 ( 属性 ) 或者 成员变量method ; // 行为 或者 成员方法}
class WashMachine{public String brand; // 品牌public String type; // 型号public double weight; // 重量public double length; // 长public double width; // 宽public double height; // 高public String color; // 颜色public void washClothes(){ // 洗衣服System.out.println("洗衣功能");}public void dryClothes(){ // 脱水System.out.println("脱水功能");}public void setTime(){ // 定时System.out.println("定时功能");}}
- 类名注意采用大驼峰定义
- 成员前写法统一为public,后面会详细解释
- 此处写的方法不带 static 关键字. 后面会详细解释
- 一般一个文件当中只定义一个类
- main方法所在的类一般要使用 public 修饰 ( 注意: Eclipse 默认会在 public 修饰的类中找 main 方法 )
- public修饰的类必须要和文件名相同
- 不要轻易去修改 public 修饰的类的名称,如果要修改,通过开发工具修改
3.类的实例化
3.1 什么是实例化
public class Dog{public String name;public String color;// 狗的属性public void barks() {System.out.println(this.name + ": 旺旺旺~~~");}// 狗的行为public void wag() {System.out.println(this.name + ": 摇尾巴~~~");}public static void main(String[] args) {Dog dogh = new Dog(); //通过new实例化对象dogh.name = "阿黄";dogh.color = "黑黄";dogh.barks();dogh.wag();Dog dogs = new Dog();dogs.name = "阿黑";dogs.color = "黑白";dogs.barks();dogs.wag();}}
运行结果如下:
- new 关键字用于创建一个对象的实例.
- 使用 . 来访问对象中的属性和方法.
- 同一个类可以创建多个实例
3.2 类和对象的说明
4. 对象的构造和初始化
4.1 构造方法
4.1.1 概念
public class Date {public int year;public int month;public int day;// 构造方法:
// 名字与类名相同,没有返回值类型,设置为void也不行
// 一般情况下使用public修饰
// 在创建对象时由编译器自动调用,并且在对象的生命周期内只调用一次public Date(int year, int month, int day){this.year = year;this.month = month;this.day = day;System.out.println("Date(int,int,int)方法被调用了");}public void printDate(){System.out.println(year + "-" + month + "-" + day);}public static void main(String[] args) {
// 此处创建了一个Date类型的对象,并没有显式调用构造方法Date d = new Date(2021,6,9); // 输出Date(int,int,int)方法被调用了d.printDate(); // 2021-6-9}
}
运行结果如下:
注意:构造方法的作用就是对对象中的成员进行初始化,并不负责给对象开辟空间。
4.1.2 特性
public class Date {public int year;public int month;public int day;// 无参构造方法public Date(){this.year = 1900;this.month = 1;this.day = 1;}// 带有三个参数的构造方法public Date(int year, int month, int day) {this.year = year;this.month = month;this.day = day;}public void printDate(){System.out.println(year + "-" + month + "-" + day);}public static void main(String[] args) {Date d = new Date(); d.printDate(); }
}
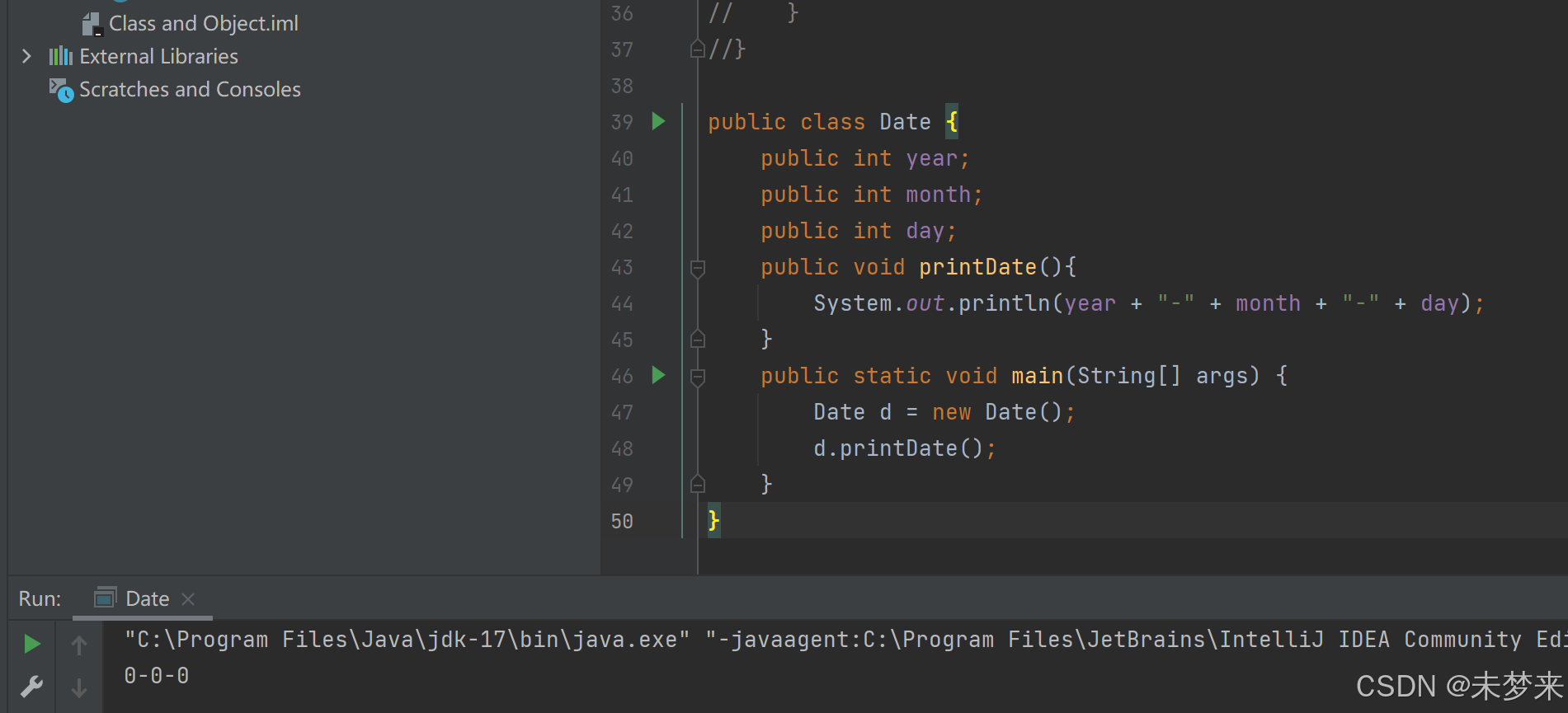
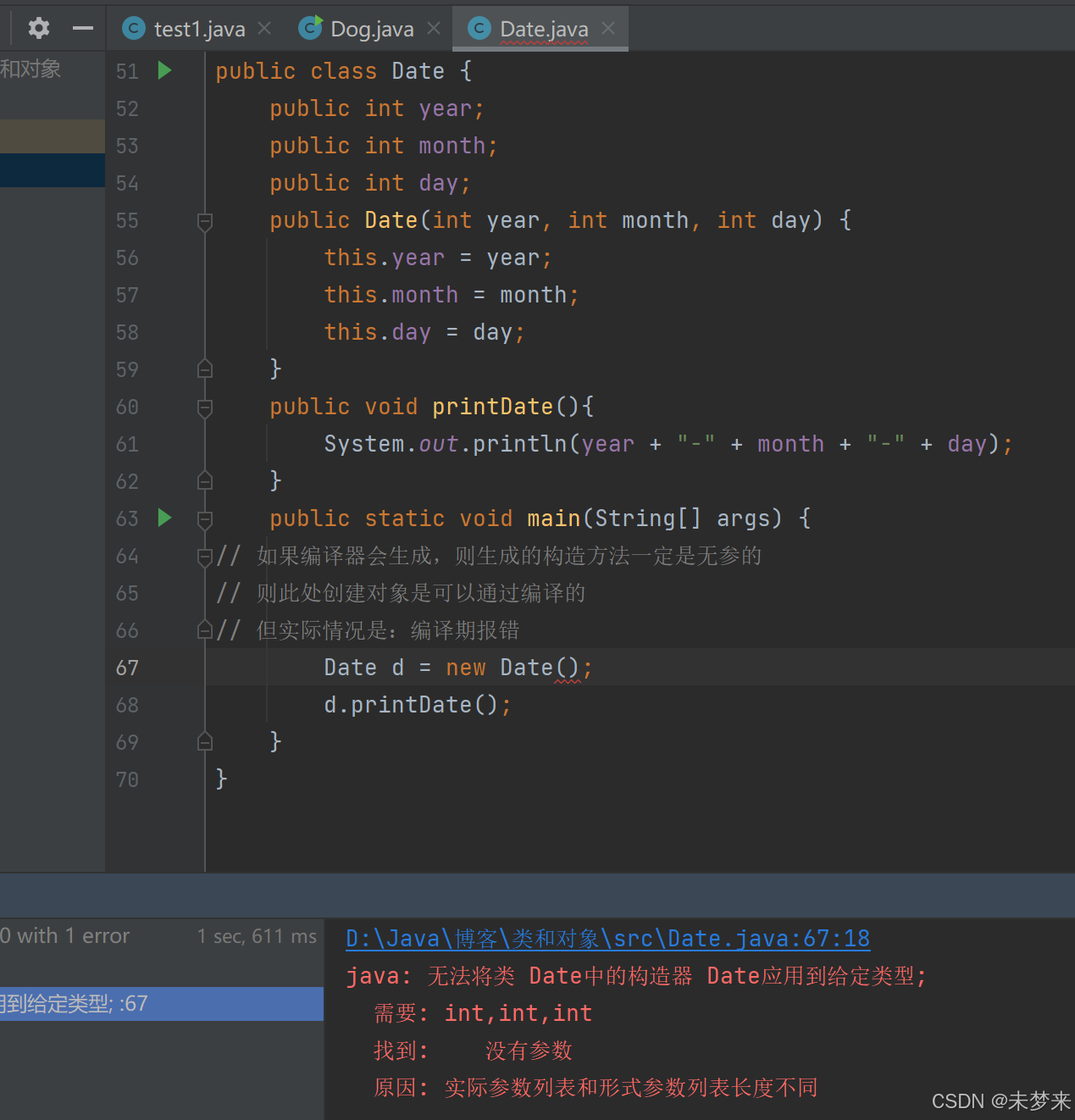
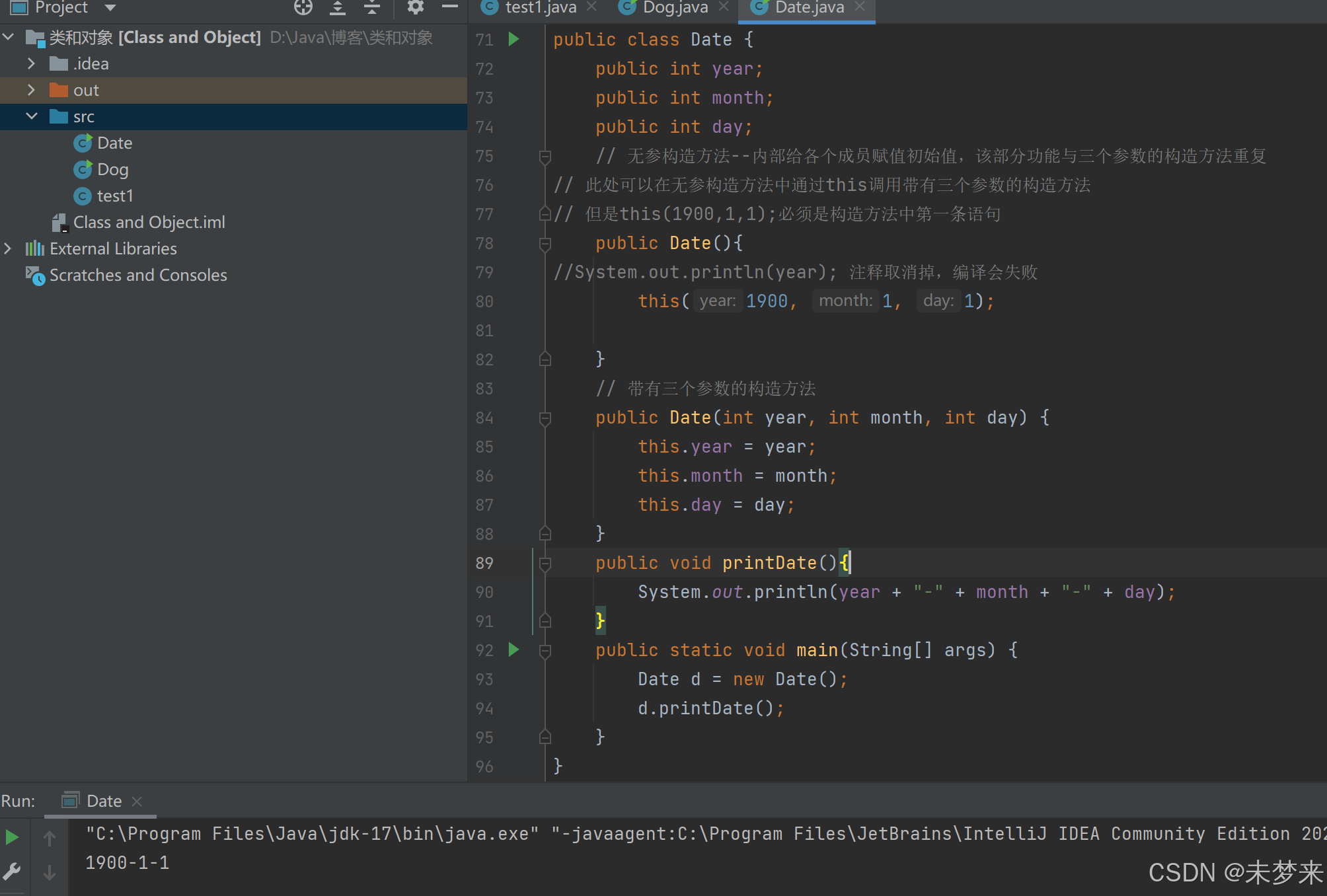
- this(...)必须是构造方法中第一条语句
- 不能形成环
7. 绝大多数情况下使用public来修饰,特殊场景下会被private修饰
4.2 this 引用
4.2.1 this 的作用
看无 this 引用的示例:
为什么代码运行结果是错误的呢?
主要原因有两个:
4.2.1 this 引用的概念
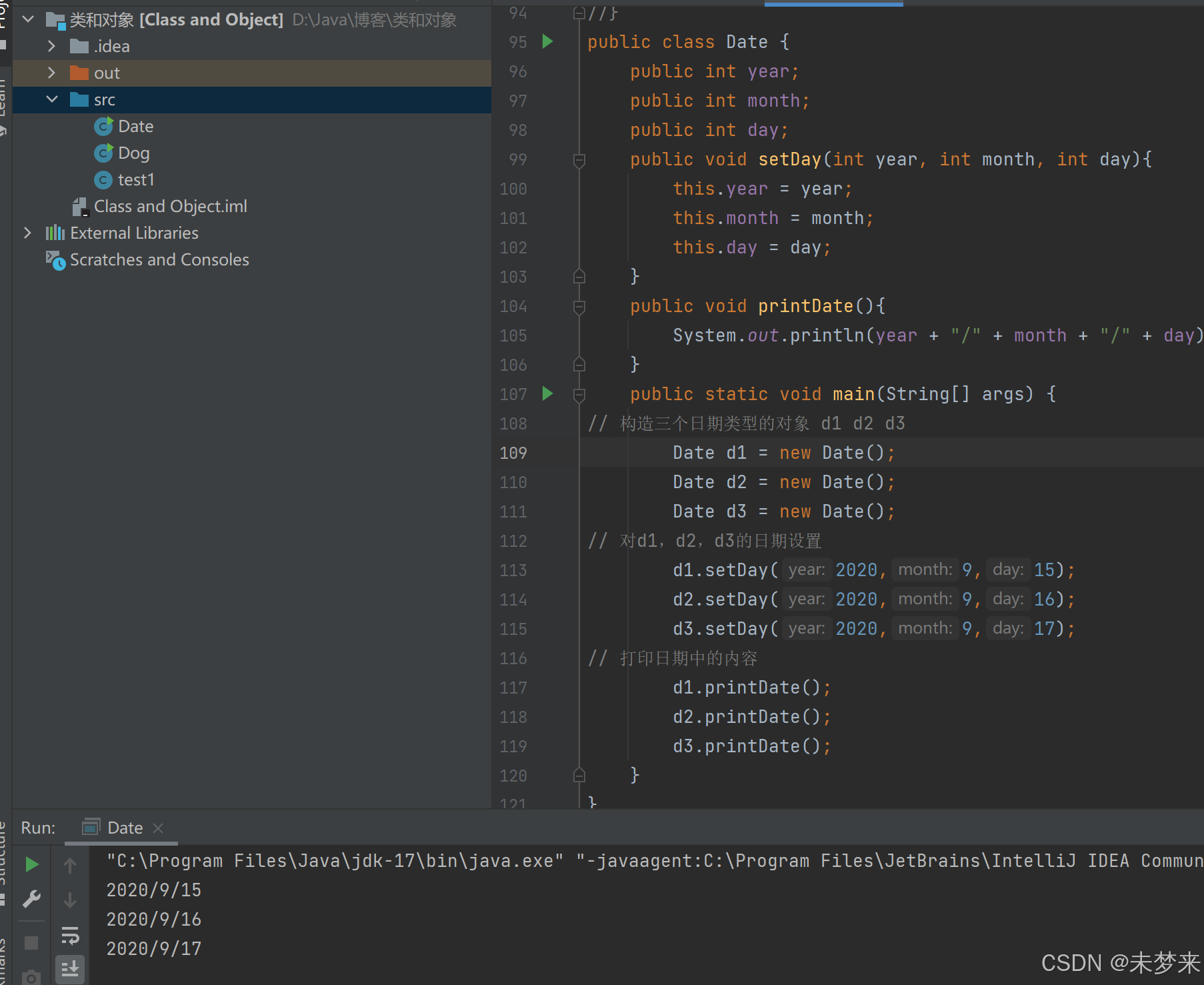
4.2.3 this 引用的特性
4.3 默认初始化
先看示例:
Date d = new Date ( 2021 , 6 , 9 );
数据类型 | 默认值 |
byte | 0 |
char | '\u0000' |
short | 0 |
int | 0 |
long | 0L |
boolean | flase |
float | 0.0f |
double | 0.0 |
reference | null |
4.4 就地初始化
在声明成员变量时,就直接给出了初始值。
代码如下:
public class Date {public int year = 1900;public int month = 1;public int day = 1;public Date(){}public Date(int year, int month, int day) {}public void printDate(){System.out.println(year + "/" + month + "/" + day);}public static void main(String[] args) {Date d1 = new Date(2021,6,9);Date d2 = new Date();d1.printDate();d2.printDate();}
}
运行结果如下:
注意:代码编译完成后,编译器会将所有给成员初始化的这些语句添加到各个构造函数中
5. static
5.1 static 修饰成员变量
代码如下:
public class Students {public String name;public String gender;public int age;public static String classRoom = "Bit306";public Students(String name,String gender,int age){this.name = name;this.gender = gender;this.age = age;}public static void main(String[] args) {System.out.println(Students.classRoom);Students s1 = new Students("Li lei", "男", 18);Students s2 = new Students("Han MeiMei", "女", 19);Students s3 = new Students("Jim", "男", 18);System.out.println(s1.classRoom);System.out.println(s2.classRoom);System.out.println(s3.classRoom);}
}
运行结果如下:
5.2 static 修饰成员方法
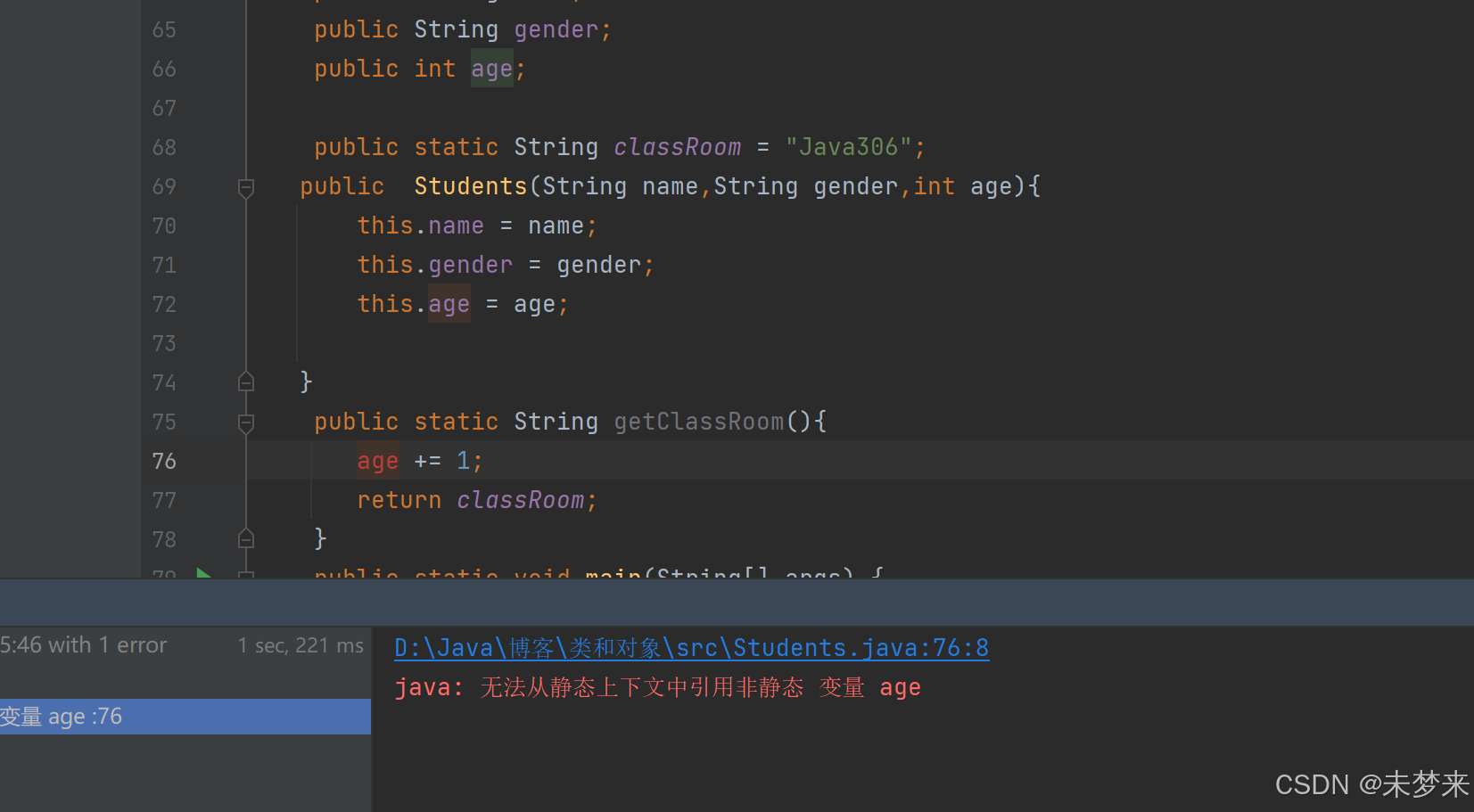
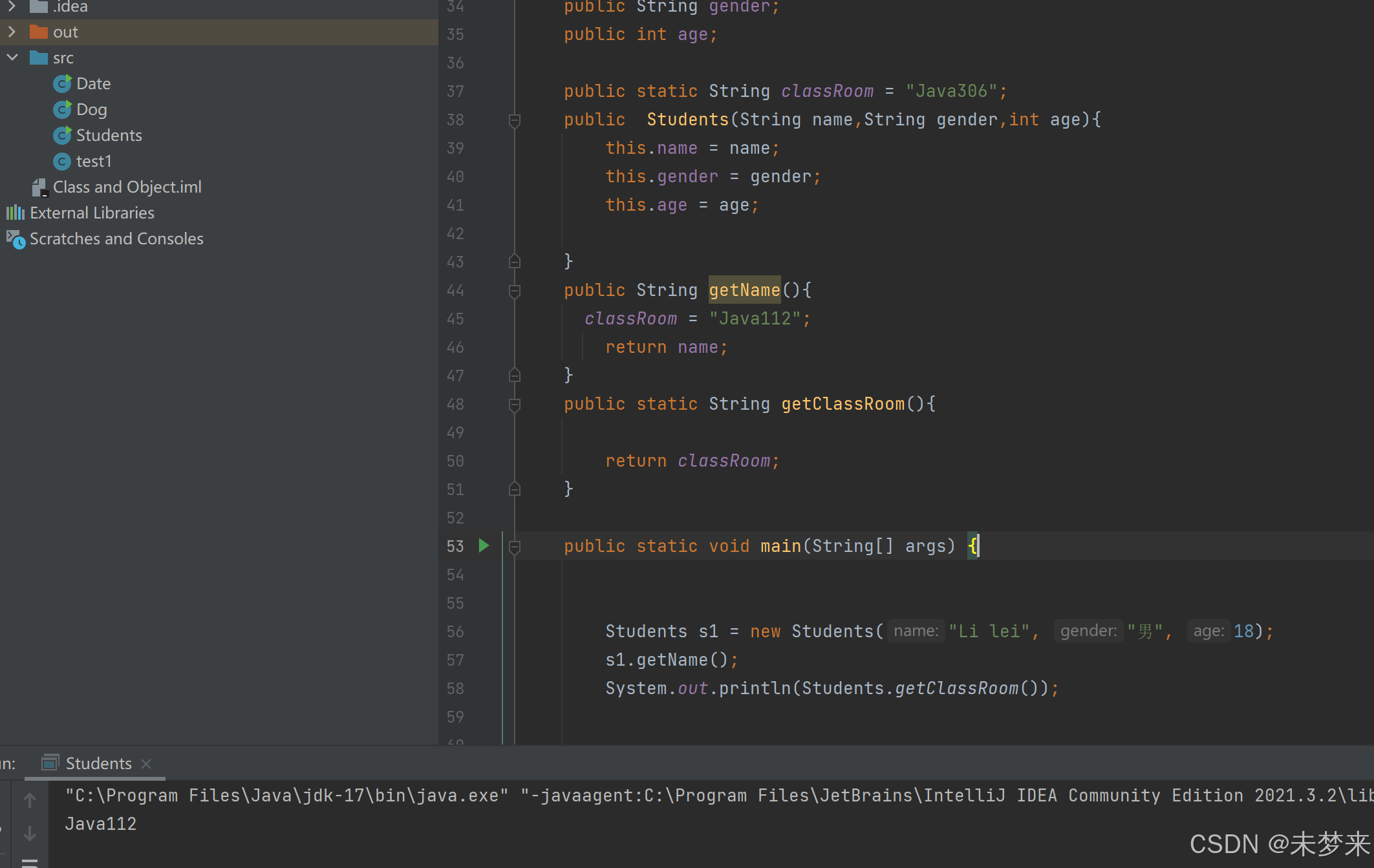
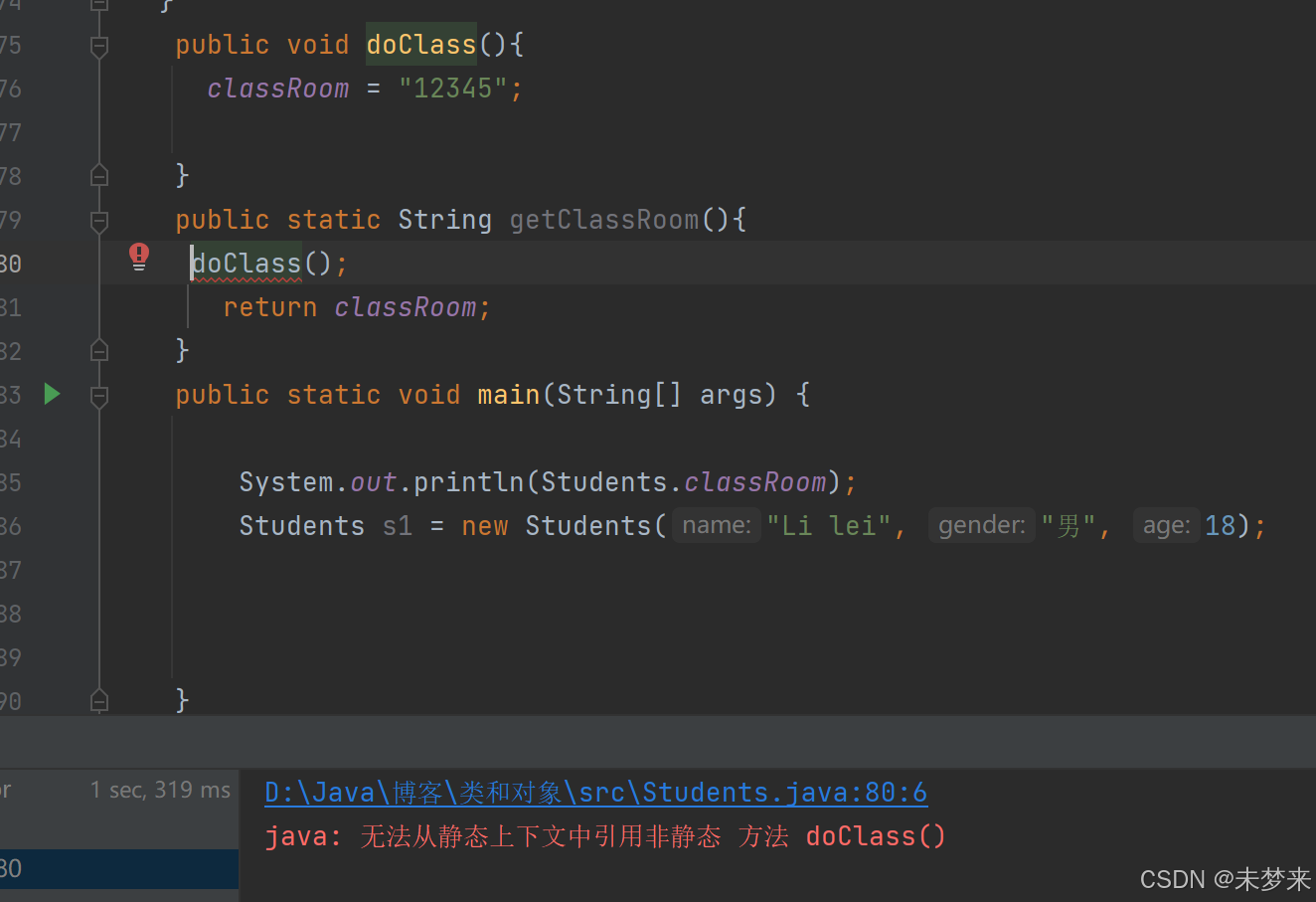
5.3 static 成员变量初始化
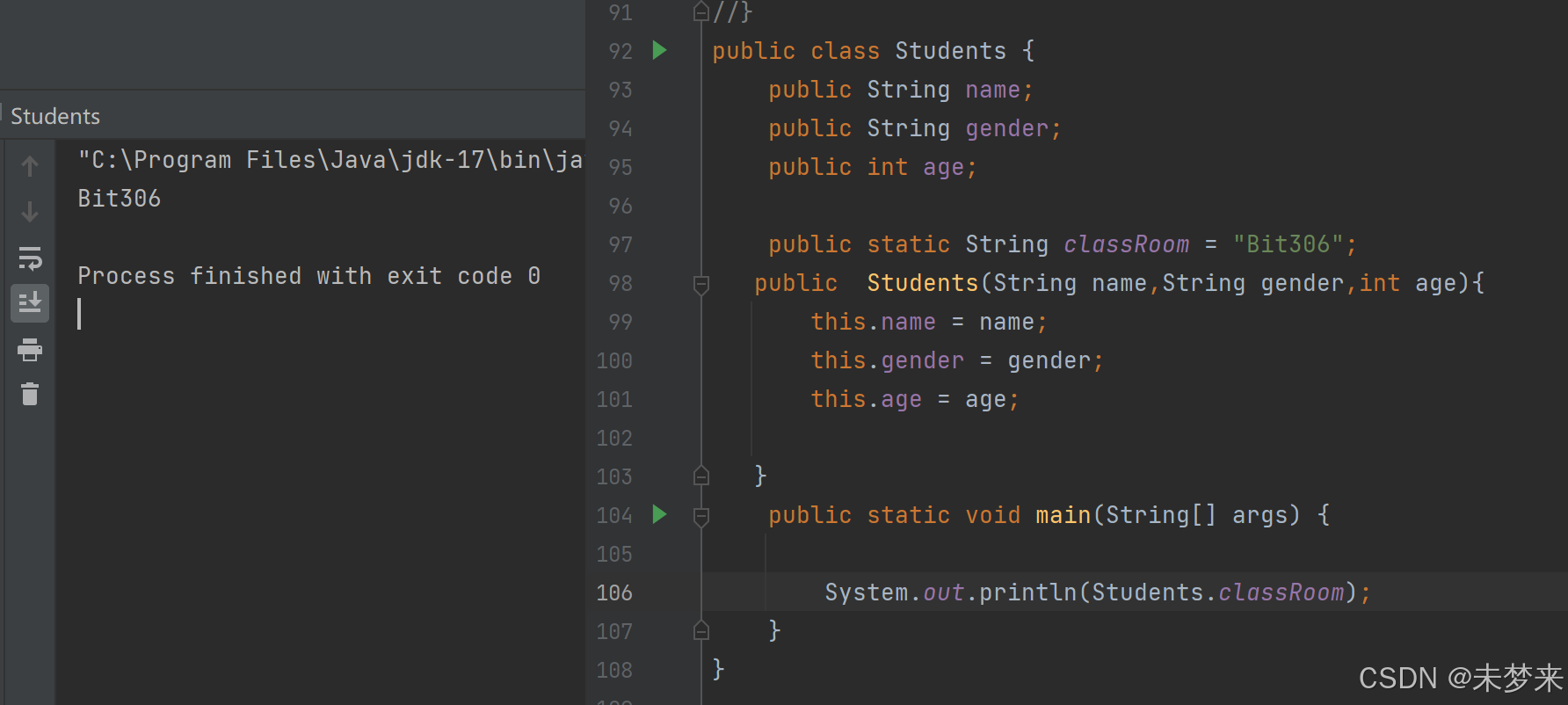
6. 代码块
6.1 代码块的概念
- 普通代码块
- 构造块
- 静态块
- 同步代码块
6.2 普通代码块
普通代码块:定义在方法中的代码块.
代码如下:
public static void main(String[] args) {{ //直接使用{}定义,普通方法块int x = 10 ;System.out.println("x1 = " +x);}int x = 100 ;System.out.println("x2 = " +x);}
运行结果如下:
注意:这种用法较少见
6.3 构造代码块
public class Students {public String name;public String gender;public int age;public double score;public Students() {System.out.println("I am Student init()!");}//实例代码块{this.name = "bit";this.age = 12;this.gender = "man";System.out.println("I am instance init()!");}public void show(){System.out.println("name: "+name+" age: "+age+" gender: "+gender);}public static void main(String[] args) {Students stu = new Students();stu.show();}
}
运行结果如下:
6.4 静态代码块
public class Students{private String name;private String gender;private int age;private double score;private static String classRoom;// 静态代码块static {classRoom = "bit306";System.out.println("I am static init()!");}public Students(){System.out.println("I am Student init()!");}public static void main(String[] args) {Students s1 = new Students();Students s2 = new Students();}
}
运行结果如下:
- 静态代码块不管生成多少个对象,其只会执行一次
- 静态成员变量是类的属性,因此是在JVM加载类时开辟空间并初始化的
- 如果一个类中包含多个静态代码块,在编译代码时,编译器会按照定义的先后次序依次执行(合并)
- 实例代码块只有在创建对象时才会执行
本文是作者学习后的总结,如果有什么不恰当的地方,欢迎大佬指正!!!
这篇关于JavaSE ——类和对象的文章就介绍到这儿,希望我们推荐的文章对编程师们有所帮助!